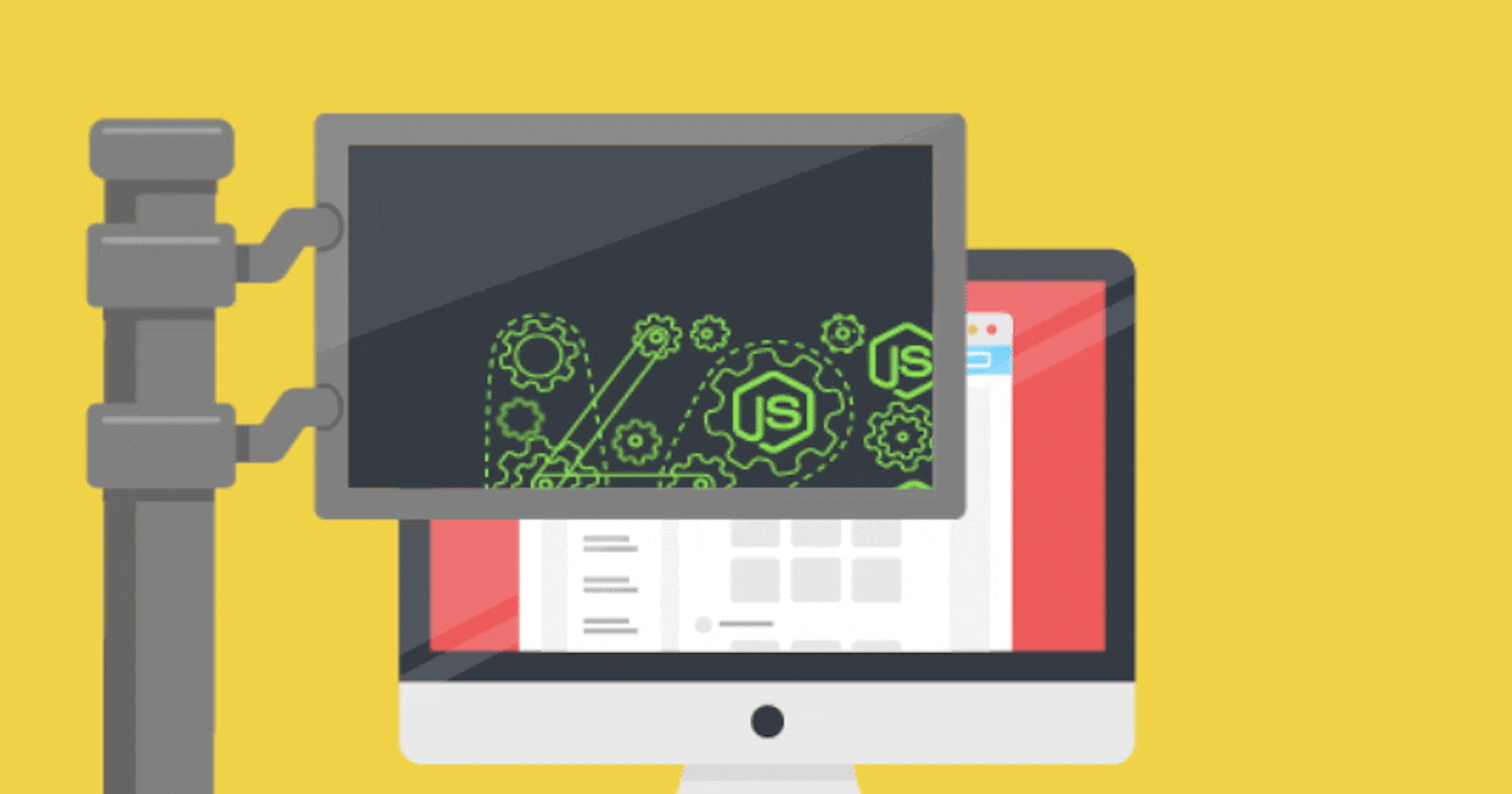
JavaScript Interview Preparation Cheat Sheet.
JavaScript Cheat Sheet(Scope, Single Thread, Call Stack, and Hoisting).
🙋 Hello! Everyone.
Today we learn about Scope
, Single Thread
, Call Stack
, and Hoisting
.
⭐Scope in JavaScript
The scope is the current context of execution in which values
and expressions are "visible" or can be referenced. If a variable
or expression is not in the current scope, it will not be available for use. Scopes can also be layered in a hierarchy, so that child scopes have access to parent scopes, but not vice versa.
JavaScript has 3 types of Scope :
- Global Scope
- Local Scope
- Block Scope
Global Scope
A variable declared at the top of a program or outside of a function is considered a global scope variable.
Let's see the example of Global Scope.
let name = "Vandit";
// Create Function
function greet(){
consol.log(`Hello! ${name}`); // Hello! Vandit
}
greet(); // Call Function
Output is:
Hello! Vandit
In the above example, a variable name
is declared at the top of a program and is a global variable. It means the variable name
can be used anywhere in the program.
The value of a global variable can be changed inside a function. For example,
let name = "Vandit";
function greet() {
name = "Hitesh";
}
// Before the Function Call
console.log(name); // Vandit
// After the Function Call
greet();
console.log(name); // Hitesh
Output is:
Vandit
Hitesh
In the above example, a variable name
is a global variable. The value of the name
is Vandit
. Then the variable name
is accessed inside a function and the value changes to Hitesh
.
Hence, the value of the name
changes after changing it inside the function.
In JavaScript, a variable can also be used without declaring it. If a variable is used without declaring it, that variable automatically becomes a global variable. For example,
function greet() {
name = "Vandit"
}
greet();
console.log(name); // Vandit
Output is:
Vandit
In the above program, the variable name
is a global variable.
If the variable was declared using let name = "Vandit"
, the program would throw an error.
Local Scope
A variable can also have a local scope, i.e it can only be accessed within a function.
Let's see the example of Local Scope.
let fName = "Hello";
function greet() {
let lName = "Vandit"
console.log(fName + lName);
}
greet();
console.log(fName + lName); // error
Output is:
Hello Vandit
console.log(fName + lName); // error
^
ReferenceError: lName is not defined
In the above program, variable fName
is a global variable and variable lName
is a local variable. The variable lName
can be accessed only inside the function greet
. Hence, when we try to access variable lName
outside of the function, an error occurs.
Block Scope
The let
keyword is block-scoped (variable can be accessed only in the immediate block).
Let's see the example of Block Scope.
// Global Variable
let fName = "Vandit";
function greet() {
// Local Variable
let lName = "Bera";
console.log(fName + " " + lName);
if (lName == "Bera") {
// Block-Scoped Variable
let country = "India";
console.log(fName + " " + lName + " " + country);
}
// variable country cannot be accessed here
console.log(fName + " " + lName + " " + country);
}
greet();
Output is:
Vandit Bera
Vandit Bera India
⭐JavaScript is a Single Thread
Javascript is a single-threaded language. This means it has one call stack and one memory heap. As expected, it executes code in order and must finish executing a piece of code before moving on to the next. It's synchronous, but at times that can be harmful.
⭐Call Stack in JavaScript
The call stack is used by JavaScript to keep track of multiple function calls. It is like a real stack in data structures where data can be pushed and popped and follows the Last In First Out (LIFO) principle. We use call stack for memorizing which function is running right now. The below example demonstrates the call stack. For example,
function fName() {
console.log("Hello! First Name");
}
function lName() {
fName();
console.log("Hello! Last Name");
}
lName();
Output is:
Hello! First Name
Hello! Last Name
Explaination:
Step 1: When the code loads in memory, the global execution context gets pushed into the stack.
Step 2: The lName() function gets called, and the execution context of lName() gets pushed into the stack.
Step 3: The execution of lName() starts and during its execution, the fName() function gets called inside the lName() function. This causes the execution context of fName() to get pushed in the call stack.
Step 4: Now the fName() function starts executing. A new stack frame of the console.log() method will be pushed to the stack.
Step 5: When the console.log() method runs, it will print “Hello! First Name” and then it will be popped from the stack. The execution context go will back to the function and now there is not any line of code that remains in the fName() function, as a result, it will also be popped from the call stack.
Step 6: This will similarly happen with the console.log() method that prints the line “Hello! Last Name” and then finally the function lName() would finish and would be pushed off the stack.
⭐Hoisting in JavaScript
Hoisting is the default behavior of moving all the declarations at the top of the scope before code execution. It gives us an advantage that no matter where functions and variables are declared, they are moved to the top of their scope regardless of whether their scope is global or local.
Hoisting in JavaScript is a behavior in which a function or a variable can be used before declaration. For example,
console.log(test); // undefined
var test;
The above program works and the output will be undefined
. The above program behaves as
var test;
console.log(test); // undefined
Since the variable test
is only declared and has no value, undefined
value is assigned to it.
Variable Hoisting
In terms of variables and constants, keyword var is hoisted and let and const does not allow hoisting. For example,
nums = 5;
console.log(nums);
var nums; // 5
In the above example, variable nums
is used before declaring it. And the program works and displays the output 5. The program behaves as:
var nums;
nums = 5;
console.log(nums); // 5
However, in JavaScript, initializations are not hoisted. For example,
console.log(nums);
var nums = 5;
Output is:
undefined
The above program behaves as:
var nums;
console.log(nums);
nums = 5;
Only the declaration is moved to the memory in the compile phase. Hence, the value of variable nums is undefined because nums is printed without initializing it.
Also, when the variable is used inside the function, the variable is hoisted only to the top of the function. For example,
var a = 4;
function greet() {
b = 'hello';
console.log(b); // hello
var b;
}
greet(); // hello
console.log(b);
Output is:
hello
console.log(b);
^
ReferenceError: b is not defined
In the above example, variable b
is hoisted to the top of the function greet
and becomes a local variable. Hence b
is only accessible inside the function. b
does not become a global variable.
If a variable is used with the let
keyword, that variable is not hoisted. For example,
a = 5;
console.log(a);
let a; // error
Output is:
a = 5;
^
ReferenceError: a is not defined
While using let
, the variable must be declared first.
Function Hoisting
A function can be called before declaring it. For example,
greet();
function greet() {
console.log('Hi, there.');
}
Output is:
Hi, there.
In the above program, the function greet
is called before declaring it and the program shows the output. This is due to hoisting.
However, when a function is used as an expression, an error occurs because only declarations are hoisted. For example;
greet();
let greet = function() {
console.log('Hi, there.');
}
Output is:
greet();
^
ReferenceError: greet is not defined
If var
was used in the above program, the error would be:
greet();
^
TypeError: greet is not a function
And that’s pretty much it. 🙂
I hope this reference guide has been helpful for you!
Thanks to:- Hitesh Chaudhary